How To: Implement Facebook Connect on the iPhone in 5 minutes from Cat Lee on Vimeo.
若想要開發一些 iPhone 小軟體,並將一些訊息發佈到 Facebook 上面,可以先逛逛這些頁面:
- Mobile - Facebook Developer Wiki
- Facebook Connect for iPhone - Facebook Developer Wiki
- Facebook Connect - Facebook Developer Wiki
- iPhone FBConnect: Facebook Connect Tutorial
剩下的就仿造 Facebook
Connect for iPhone - Facebook Developer Wiki 所提到的設定,先到 facebook's facebook-iphone-sdk at master - GitHub 下載相關程式碼,可在右邊直接按 Download Source 下載。

以下參考 iPhone 開發教學 - 環境設置和第一支程式 Hello World 範例,建立一個簡單的範例後,接著去打開下載回來的檔案,此例為 facebook-facebook-iphone-sdk-5382dcd.zip ,並且解開後找其子目錄 src 中,點選開啟 FBConnect.xcodeproj ,接著將 FBConnect 拖拉到 HelloWorld 上頭,並且使用預設選項不勾選 copy items 囉
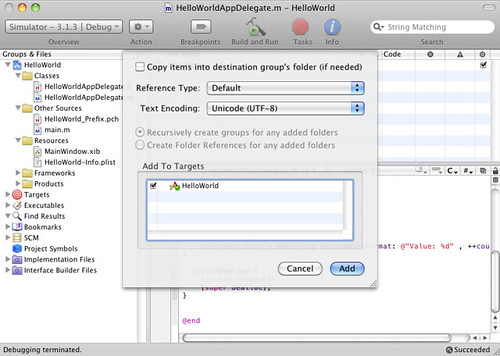
接著,設定 HelloWorld 這個專案,[Xcode]->[Project]->[Edit Project Setting]->[Build]->[Search Path]->[Headers Search Paths] 點選右邊空白,增加你下載的 facebook-iphone-sdk 程式碼的位置,在此使用 /Users/uid/Downloads/facebook-facebook-iphone-sdk-5382dcd/src,弄完接著就可以對 HelloWorld 進行編譯看看,[Xcode]->[Project]->[Build] ,在此只看到一個 warning ,先暫時不管他。
開始正式測試 Facebook 與 iPhone 的結合啦
原樣:
HelloWorldAppDelegate.h
#import <UIKit/UIKit.h>
@interface HelloWorldAppDelegate : NSObject <UIApplicationDelegate> {
UIWindow *window;
IBOutlet UILabel *showLabel;
IBOutlet UIButton *actionButton;
}
@property (nonatomic, retain) IBOutlet UIWindow *window;
- (IBAction)updateLabel;
@end
@interface HelloWorldAppDelegate : NSObject <UIApplicationDelegate> {
UIWindow *window;
IBOutlet UILabel *showLabel;
IBOutlet UIButton *actionButton;
}
@property (nonatomic, retain) IBOutlet UIWindow *window;
- (IBAction)updateLabel;
@end
HelloWorldAppDelegate.m
#import "HelloWorldAppDelegate.h"
@implementation HelloWorldAppDelegate
@synthesize window;
- (void)applicationDidFinishLaunching:(UIApplication *)application {
// Override point for customization after application launch
[window makeKeyAndVisible];
}
- (void)awakeFromNib {
showLabel.text = @"Hello World!";
}
- (IBAction)updateLabel {
static int count = 0;
showLabel.text = [NSString stringWithFormat: @"Value: %d" , ++count];
}
- (void)dealloc {
[window release];
[super dealloc];
}
@end
@implementation HelloWorldAppDelegate
@synthesize window;
- (void)applicationDidFinishLaunching:(UIApplication *)application {
// Override point for customization after application launch
[window makeKeyAndVisible];
}
- (void)awakeFromNib {
showLabel.text = @"Hello World!";
}
- (IBAction)updateLabel {
static int count = 0;
showLabel.text = [NSString stringWithFormat: @"Value: %d" , ++count];
}
- (void)dealloc {
[window release];
[super dealloc];
}
@end
更改後:
HelloWorldAppDelegate.h
#import <UIKit/UIKit.h>
#import "FBConnect/FBConnect.h"
#import "FBConnect/FBSession.h"
#define FB_APP_KEY @"YOUR_APP_KEY"
#define FB_SEC_KEY @"YOU_SEC_KEY"
@interface HelloWorldAppDelegate : NSObject <UIApplicationDelegate> {
UIWindow *window;
IBOutlet UILabel *showLabel;
IBOutlet UIButton *actionButton;
IBOutlet FBLoginButton *fbButton;
FBSession *fbSession;
id<FBRequestDelegate> delegate;
int status;
}
@property (nonatomic, retain) IBOutlet UIWindow *window;
@property (nonatomic, retain) IBOutlet FBLoginButton *fbButton;
@property (nonatomic, retain) FBSession *fbSession;
@property(nonatomic,assign) id<FBRequestDelegate> delegate;
- (IBAction)updateLabel;
@end
#import "FBConnect/FBConnect.h"
#import "FBConnect/FBSession.h"
#define FB_APP_KEY @"YOUR_APP_KEY"
#define FB_SEC_KEY @"YOU_SEC_KEY"
@interface HelloWorldAppDelegate : NSObject <UIApplicationDelegate> {
UIWindow *window;
IBOutlet UILabel *showLabel;
IBOutlet UIButton *actionButton;
IBOutlet FBLoginButton *fbButton;
FBSession *fbSession;
id<FBRequestDelegate> delegate;
int status;
}
@property (nonatomic, retain) IBOutlet UIWindow *window;
@property (nonatomic, retain) IBOutlet FBLoginButton *fbButton;
@property (nonatomic, retain) FBSession *fbSession;
@property(nonatomic,assign) id<FBRequestDelegate> delegate;
- (IBAction)updateLabel;
@end
HelloWorldAppDelegate.m
#import "HelloWorldAppDelegate.h"
@implementation HelloWorldAppDelegate
@synthesize window;
@synthesize fbButton;
@synthesize fbSession;
@synthesize delegate;
- (void)applicationDidFinishLaunching:(UIApplication *)application {
status = 0 ;
// Override point for customization after application launch
[window makeKeyAndVisible];
}
- (void)awakeFromNib {
showLabel.text = @"Hello World!";
if ( self.fbSession == nil ) {
self.fbSession = [FBSession sessionForApplication:FB_APP_KEY secret:FB_SEC_KEY delegate:self ];
}
}
- (void)session:(FBSession*)session didLogin:(FBUID)uid {
status = 1;
NSString* fql = [NSString stringWithFormat:@"select uid,name from user where uid == %lld",self.fbSession.uid];
NSDictionary* params = [NSDictionary dictionaryWithObject:fql forKey:@"query"];
[[FBRequest requestWithDelegate:self] call:@"facebook.fql.query" params:params];
}
- (void)request:(FBRequest*)request didLoad:(id)result {
if ([request.method isEqualToString:@"facebook.fql.query"]) {
NSString * username = [ ((NSDictionary*)[(NSArray*)result objectAtIndex:0 ]) objectForKey:@"name"] ;
showLabel.text = [NSString stringWithFormat:@"Hi, %@" , username ];
}
}
- (IBAction)updateLabel {
if ( status == 0 ) { // do login
FBLoginDialog* dialog = [[[FBLoginDialog alloc] initWithSession:self.fbSession] autorelease];
[dialog show];
}
else { // do post to Wall
FBStreamDialog* dialog = [[[FBStreamDialog alloc] init] autorelease];
dialog.delegate = self;
dialog.userMessagePrompt = @"Example prompt";
dialog.attachment = @
"{"
"\"name\":\"Facebook Connect for iPhone\","
"\"href\":\"http://developers.facebook.com/connect.php?tab=iphone\","
"\"caption\":\"Caption\","
"\"description\":\"Description\","
"\"media\":[{"
"\"type\":\"image\","
"\"src\":\"http://img40.yfrog.com/img40/5914/iphoneconnectbtn.jpg\","
"\"href\":\"http://developers.facebook.com/connect.php?tab=iphone/\"}],"
"\"properties\":{"
"\"another link\":{"
"\"text\":\"Facebook home page\","
"\"href\":\"http://www.facebook.com\""
"}"
"}"
"}";
// dialog.targetId = @"999999"; // replace this with a friend's UID
[dialog show];
}
}
- (void)dealloc {
[self.fbButton release];
[self.fbSession release];
[window release];
[super dealloc];
}
@end
@implementation HelloWorldAppDelegate
@synthesize window;
@synthesize fbButton;
@synthesize fbSession;
@synthesize delegate;
- (void)applicationDidFinishLaunching:(UIApplication *)application {
status = 0 ;
// Override point for customization after application launch
[window makeKeyAndVisible];
}
- (void)awakeFromNib {
showLabel.text = @"Hello World!";
if ( self.fbSession == nil ) {
self.fbSession = [FBSession sessionForApplication:FB_APP_KEY secret:FB_SEC_KEY delegate:self ];
}
}
- (void)session:(FBSession*)session didLogin:(FBUID)uid {
status = 1;
NSString* fql = [NSString stringWithFormat:@"select uid,name from user where uid == %lld",self.fbSession.uid];
NSDictionary* params = [NSDictionary dictionaryWithObject:fql forKey:@"query"];
[[FBRequest requestWithDelegate:self] call:@"facebook.fql.query" params:params];
}
- (void)request:(FBRequest*)request didLoad:(id)result {
if ([request.method isEqualToString:@"facebook.fql.query"]) {
NSString * username = [ ((NSDictionary*)[(NSArray*)result objectAtIndex:0 ]) objectForKey:@"name"] ;
showLabel.text = [NSString stringWithFormat:@"Hi, %@" , username ];
}
}
- (IBAction)updateLabel {
if ( status == 0 ) { // do login
FBLoginDialog* dialog = [[[FBLoginDialog alloc] initWithSession:self.fbSession] autorelease];
[dialog show];
}
else { // do post to Wall
FBStreamDialog* dialog = [[[FBStreamDialog alloc] init] autorelease];
dialog.delegate = self;
dialog.userMessagePrompt = @"Example prompt";
dialog.attachment = @
"{"
"\"name\":\"Facebook Connect for iPhone\","
"\"href\":\"http://developers.facebook.com/connect.php?tab=iphone\","
"\"caption\":\"Caption\","
"\"description\":\"Description\","
"\"media\":[{"
"\"type\":\"image\","
"\"src\":\"http://img40.yfrog.com/img40/5914/iphoneconnectbtn.jpg\","
"\"href\":\"http://developers.facebook.com/connect.php?tab=iphone/\"}],"
"\"properties\":{"
"\"another link\":{"
"\"text\":\"Facebook home page\","
"\"href\":\"http://www.facebook.com\""
"}"
"}"
"}";
// dialog.targetId = @"999999"; // replace this with a friend's UID
[dialog show];
}
}
- (void)dealloc {
[self.fbButton release];
[self.fbSession release];
[window release];
[super dealloc];
}
@end
一開始
按下按鈕,進入登入畫面
登入後,顯示暱稱
按下按鈕,進入發佈到塗鴉牆的過程
輸入資料中
在 Facebook 上的顯示
程式解說:
透過 status 變數控制,當 status ==0 時按下 button 時,歸類在做 login 的動作,等到登入成功後,則 status 更新為 1 ,當 status == 1 時,按下 button 後,則進入發佈到塗鴉牆的動作。
目前程式有點類似拼湊起來的,編譯上會有 warning:
Class 'HelloWorldAppDelegate' does not implement the 'FBSesionDelegate' protocol
Class 'HelloWorldAppDelegate' does not implement the 'FBRequestDelegate'
protocol
Class 'HelloWorldAppDelegate' does not implement the 'FBDialogDelegate'
protocol
Class 'HelloWorldAppDelegate' does not implement the 'FBRequestDelegate'
protocol
Class 'HelloWorldAppDelegate' does not implement the 'FBDialogDelegate'
protocol
建議可以看看 iPhone FBConnect: Facebook Connect Tutorial 所規劃的程式範例,上頭有作架構上的切割,在此只是純粹測試 Facebook API ,除了全部擠在一起外,還有些函數寫的不謹慎,只是恰好此情況不會出現什麼問題囉!
小小的應用看起來真是複雜~ 真是讓我卻步... Orz
回覆刪除版主回覆:(11/28/2010 02:22:20 AM)
哈哈, 我多麼希望可以不用 Mac OS 環境就能寫出來, 這應該是我感到最大的困難點, 還有 framework 的甜蜜包袱 orz
請問一下 我要怎麼把按ㄉ那個按鈕 變成F的圖案阿?
回覆刪除請問我要如何用xcode 寫Facebook 程式?
回覆刪除我想寫可以發表狀態至fb上的app...
然後狀態下面有來自xxxx這樣
謝謝
版主回覆:(03/22/2010 08:55:11 AM)
fb sdk 更新、變化很快,這些筆記大概都不適用了,建議直接看 facebook 官網的介紹吧 :)